1.Firebase與專案連結
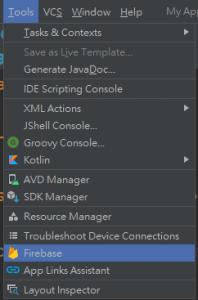
2.點擊Storage將library與google-services.json導入進專案
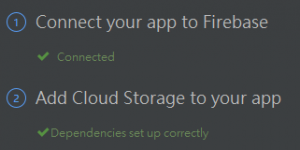
3.修改Stroage規則
service firebase.storage {
match /b/{bucket}/o {
match /{allPaths=**} {
allow read, write;
}
}
}
4.上傳檔案到Storage
//設定路徑與檔名
val reference = FirebaseStorage.getInstance().reference
val girlRef = reference.child("images/girl.jpg")
val girl2Ref = reference.child("images/girl2.jpg")
//將圖片換成byteArray
//第一張
val girl = ContextCompat.getDrawable(this, R.drawable.girl)!!.toBitmap()
val baos = ByteArrayOutputStream()
girl.compress(Bitmap.CompressFormat.JPEG, 100, baos)
val girlData = baos.toByteArray()
//第二張
val baos2 = ByteArrayOutputStream()
val girl2 = ContextCompat.getDrawable(this, R.drawable.girl2)!!.toBitmap()
girl2.compress(Bitmap.CompressFormat.JPEG, 100, baos2)
val girl2Data = baos2.toByteArray()
//上傳到Firebase(有分Bytes,File,Stream),並取得圖片網址
girlRef.putBytes(girlData)
.addOnSuccessListener {
Toast.makeText(this, "上傳成功", Toast.LENGTH_SHORT).show()
}.addOnFailureListener {
Toast.makeText(this, "上傳失敗", Toast.LENGTH_SHORT).show()
}.continueWithTask {
girlRef.downloadUrl
}.addOnCompleteListener { task ->
if (task.isSuccessful) {
val downloadUri = task.result
}
}
girl2Ref.putBytes(girl2Data)
.addOnSuccessListener {
Toast.makeText(this, "上傳成功", Toast.LENGTH_SHORT).show()
}.addOnFailureListener {
Toast.makeText(this, "上傳失敗", Toast.LENGTH_SHORT).show()
}.continueWithTask {
girl2Ref.downloadUrl
}.addOnCompleteListener { task ->
if (task.isSuccessful) {
val downloadUri = task.result
}
}

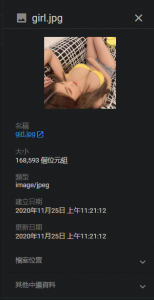
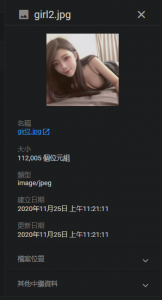
Girl
Girl2
5.利用Glide讀取網址顯示圖片
導入Glide Library
implementation 'com.github.bumptech.glide:glide:4.11.0'
在callback網址的地方使用Glide
continueWithTask {
girlRef.downloadUrl
}.addOnCompleteListener { task ->
if (task.isSuccessful) {
val downloadUri = task.result
Glide.with(this)
.load(downloadUri)
.into(photo)
}
}
continueWithTask {
girl2Ref.downloadUrl
}.addOnCompleteListener { task ->
if (task.isSuccessful) {
val downloadUri = task.result
Glide.with(this)
.load(downloadUri)
.into(photo1)
}
}
6.效果展示
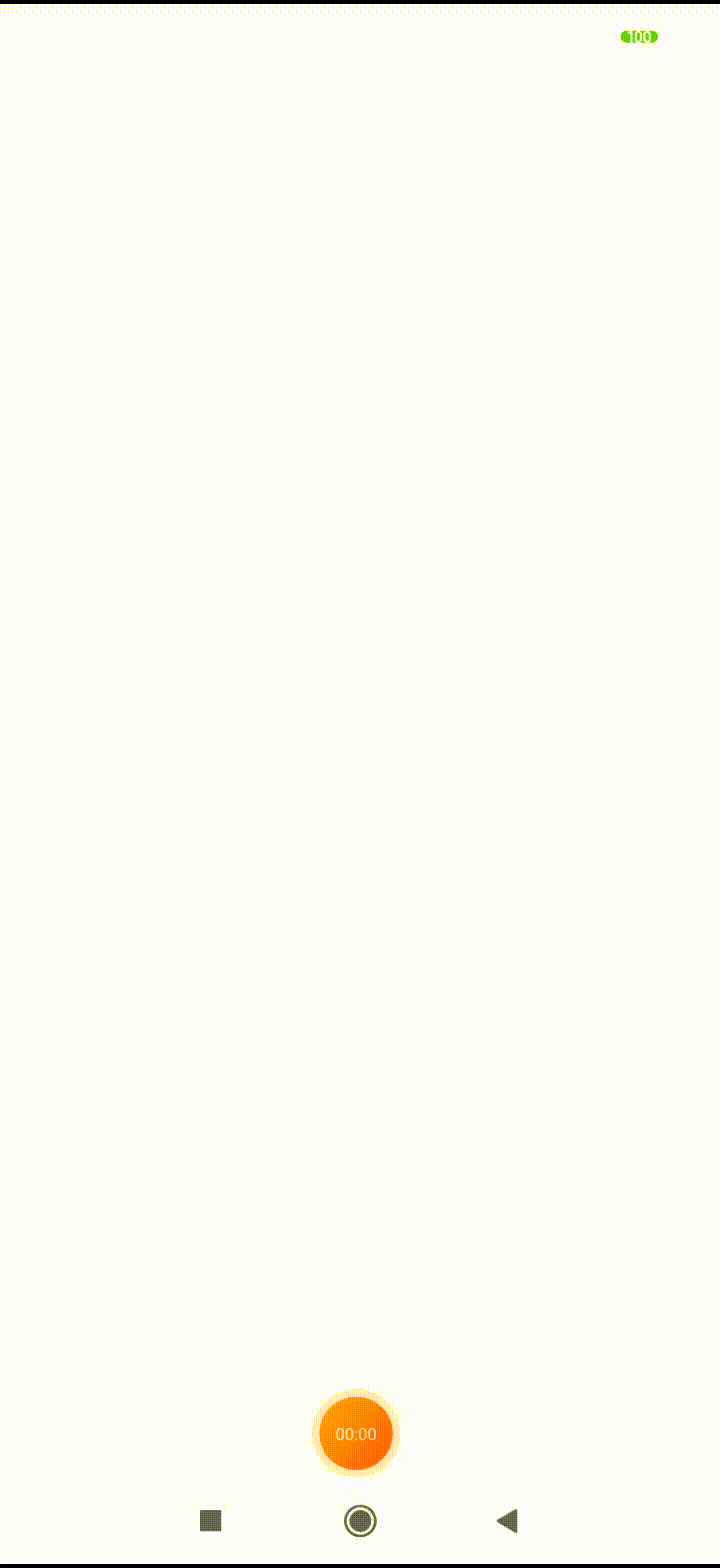