Android PopupWindow 彈出客製化視窗
PopupWindow應用的場景還是蠻多的,顯示在activity介面之上的任意彈出視窗基本上都可以用PopupWindow來實現。因為它可以顯示任意的View生成一个PopupWindow,最基本的三個條件是一定要設置的contentView,width,height。
文章目錄
- 自定義要顯示的Layout
- 創建PopupWindow
- PopupWindow添加動畫
- PopupWindow顯示視窗位置
- 程式範例
- 效果展示
1.自定義要顯示的Layout
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="wrap_content">
<androidx.constraintlayout.widget.ConstraintLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginStart="32dp"
android:layout_marginEnd="32dp"
android:background="#FF00FF"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent">
<TextView
android:id="@+id/a"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="32dp"
android:layout_marginEnd="32dp"
android:background="#FF0000"
android:gravity="center"
android:text="A"
android:textColor="@color/black"
android:textSize="30sp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<TextView
android:id="@+id/b"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:background="#FFFF00"
android:gravity="center"
android:text="B"
android:textColor="@color/black"
android:textSize="30sp"
app:layout_constraintEnd_toEndOf="@+id/a"
app:layout_constraintStart_toStartOf="@+id/a"
app:layout_constraintTop_toBottomOf="@+id/a" />
</androidx.constraintlayout.widget.ConstraintLayout>
</androidx.constraintlayout.widget.ConstraintLayout>
2.創建PopupWindow(必要三個參數)
val popupWindow = PopupWindow(this).apply {
contentView = myContentView
width = ViewGroup.LayoutParams.MATCH_PARENT
height = ViewGroup.LayoutParams.WRAP_CONTENT
}
3.PopupWindow添加動畫
創建anim資料夾
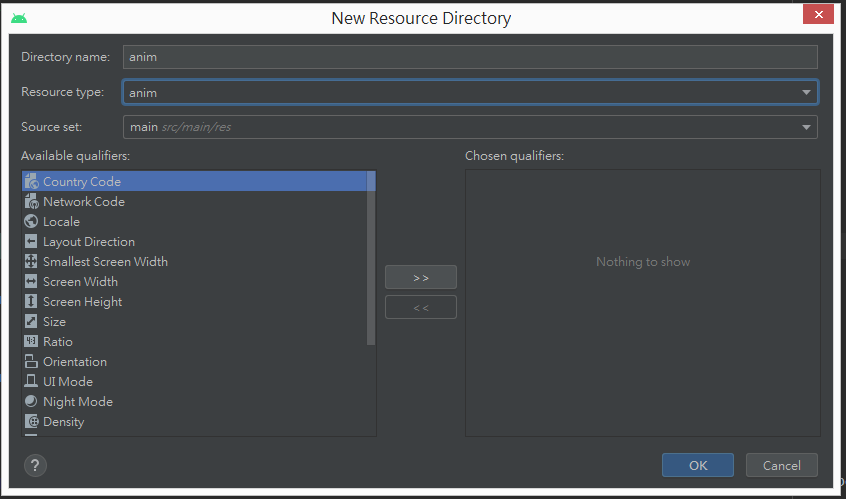
創建開啟動畫
<set xmlns:android="http://schemas.android.com/apk/res/android">
<scale
android:duration="500"
android:fromXScale="0.5"
android:fromYScale="0.5"
android:pivotX="50%"
android:pivotY="50%"
android:toXScale="1.0"
android:toYScale="1.0" />
<alpha
android:duration="500"
android:fromAlpha="0.0"
android:toAlpha="1.0" />
</set>
創建關閉動畫
<set xmlns:android="http://schemas.android.com/apk/res/android">
<scale
android:duration="500"
android:fromXScale="1.0"
android:fromYScale="1.0"
android:pivotX="50%"
android:pivotY="50%"
android:toXScale="0.5"
android:toYScale="0.5" />
<alpha
android:duration="500"
android:fromAlpha="1.0"
android:toAlpha="0.0" />
</set>
添加到Style
<resources xmlns:tools="http://schemas.android.com/tools">
<style name="PopupAnimation" parent="android:Animation">
<item name="android:windowEnterAnimation">@anim/popup_enter</item>
<item name="android:windowExitAnimation">@anim/popup_exit</item>
</style>
</resources>
4.PopupWindow顯示視窗位置
//自行設定位置
popupWindow.showAtLocation(view, Gravity.NO_GRAVITY, button.x.toInt(), button.y.toInt() - popupHeight)
//點擊View的下方
popupWindow.showAsDropDown(view)
5.程式範例
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
val button = findViewById<Button>(R.id.button)
button.setOnClickListener { view ->
val myContentView = layoutInflater.inflate(R.layout.my_pop, null)
myContentView.measure(MeasureSpec.UNSPECIFIED, MeasureSpec.UNSPECIFIED);
val popupHeight = myContentView.measuredHeight
val popupWindow = PopupWindow(this).apply {
contentView = myContentView
width = ViewGroup.LayoutParams.MATCH_PARENT
height = ViewGroup.LayoutParams.WRAP_CONTENT
animationStyle = R.style.PopupAnimation
//沒添加會一直創建新的
isFocusable = true
//透明背景
setBackgroundDrawable(ColorDrawable(Color.TRANSPARENT))
}
myContentView.findViewById<TextView>(R.id.a).setOnClickListener {
Toast.makeText(this, "A", Toast.LENGTH_SHORT).show()
}
myContentView.findViewById<TextView>(R.id.b).setOnClickListener {
Toast.makeText(this, "B", Toast.LENGTH_SHORT).show()
}
popupWindow.showAtLocation(view, Gravity.NO_GRAVITY, button.x.toInt(), button.y.toInt() - popupHeight)
//popupWindow.showAsDropDown(view)
}
}
}
6.效果展示
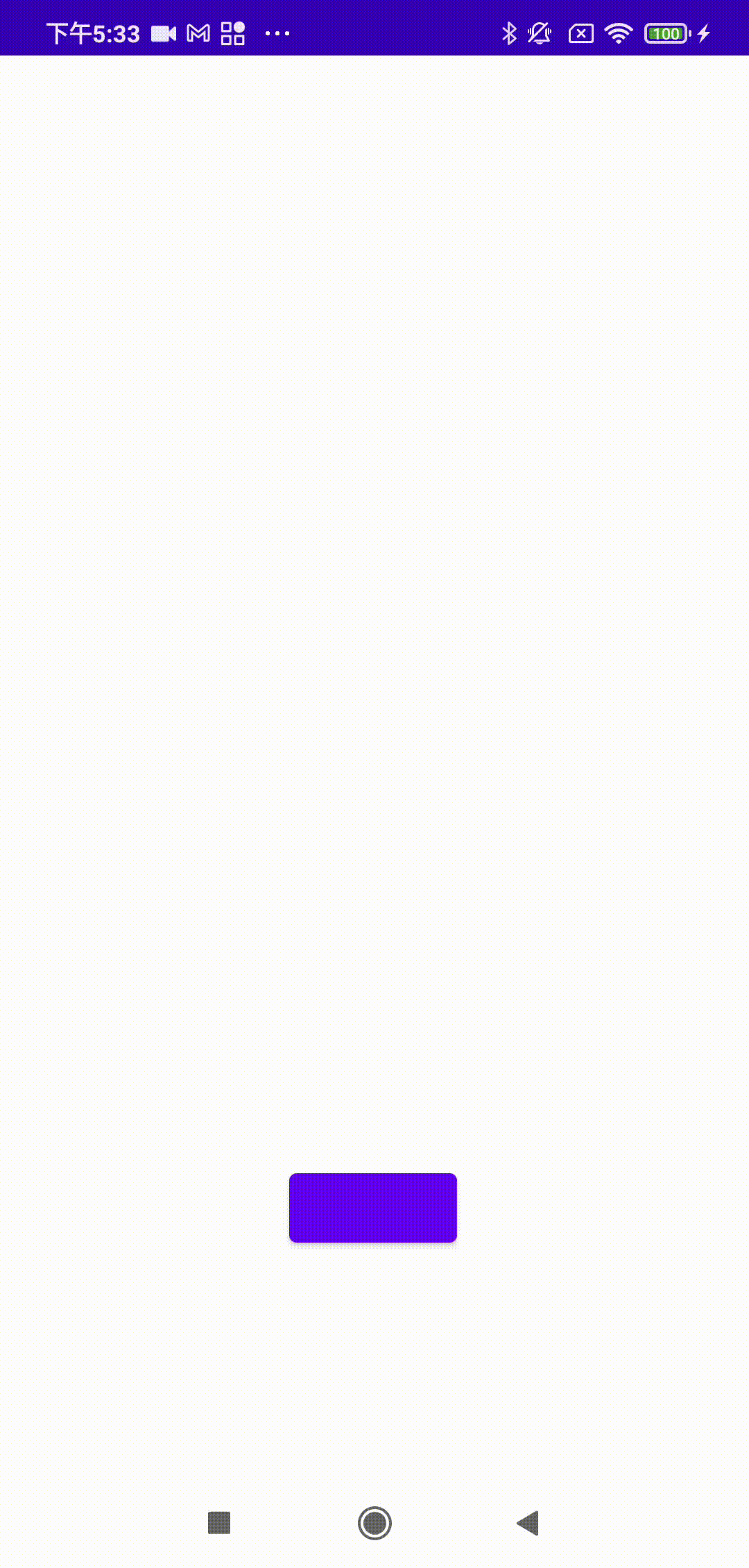