【Material Design】Android Top app bar 頂部應用欄 範例
Android top app bar 是一個專門為 Android 裝置設計的應用程式狀態列,它包含一些按鈕,根據使用者行為定制配置功能,以滿足應用程式和歡迎使用者的需求。
此頂部標題欄會出現在手機和平板電腦顯示區域的頂部,可提供應用程式導覽,快速訪問和功能按鈕,以及重要應用程式功能和相關特性的導航。

文章目錄
- Top app bar 滑動上抬應用欄
- Top app bar 滑動上抬標題與應用欄平行
- Top app bar 滑動上抬標題與應用欄平行 + 圖片
- Top app bar 操作欄
- Developer Documents Top app bar
1.Top app bar 滑動上抬應用欄
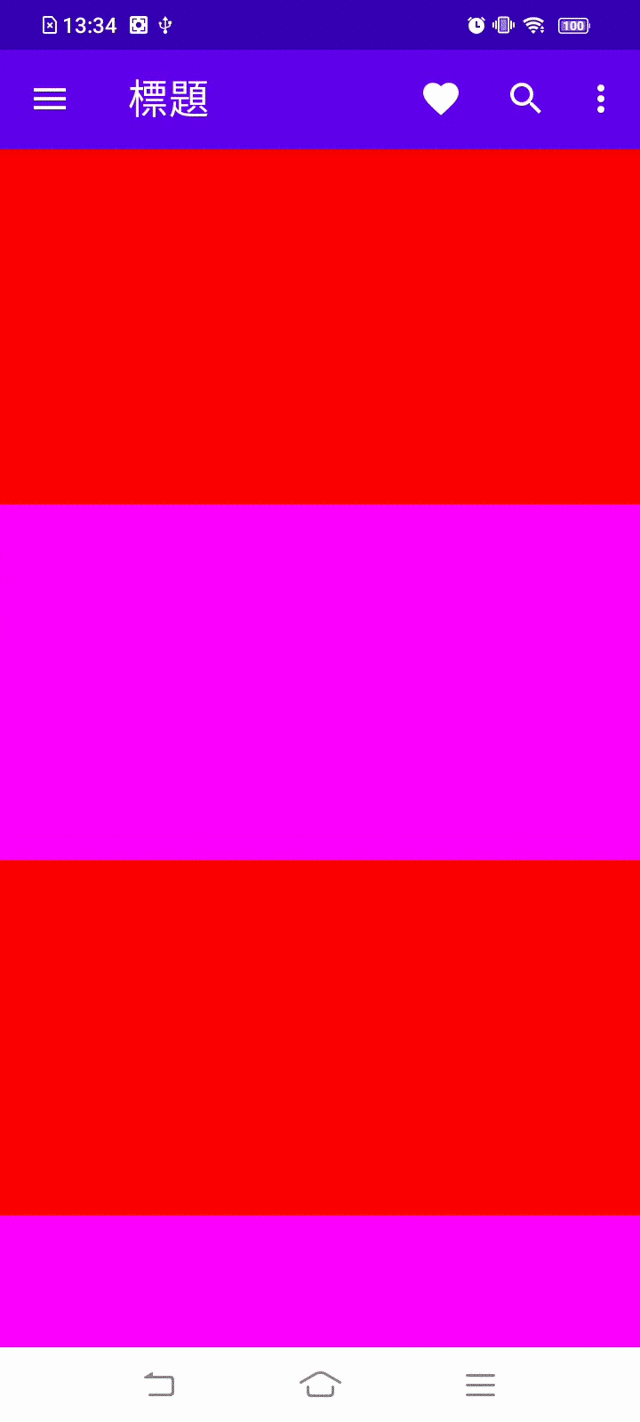
menu/top_app_bar.xml
<?xml version="1.0" encoding="utf-8"?>
<menu xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto">
<item
android:id="@+id/favorite"
android:icon="@drawable/ic_favorite_24dp"
android:title="favorite"
app:showAsAction="ifRoom" />
<item
android:id="@+id/search"
android:icon="@drawable/ic_search_24dp"
android:title="search"
app:showAsAction="ifRoom" />
<item
android:id="@+id/more"
android:title="more"
app:showAsAction="never" />
</menu>
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<layout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools">
<data>
</data>
<androidx.coordinatorlayout.widget.CoordinatorLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<com.google.android.material.appbar.AppBarLayout
android:id="@+id/appBarLayout"
android:layout_width="match_parent"
android:layout_height="wrap_content">
<com.google.android.material.appbar.MaterialToolbar
android:id="@+id/topAppBar"
style="@style/Widget.MaterialComponents.Toolbar.Primary"
android:layout_width="match_parent"
android:layout_height="?attr/actionBarSize"
app:layout_scrollFlags="scroll|enterAlways|snap"
app:menu="@menu/top_app_bar"
app:navigationIcon="@drawable/ic_menu_24dp"
app:title="標題" />
</com.google.android.material.appbar.AppBarLayout>
<androidx.core.widget.NestedScrollView
android:layout_width="match_parent"
android:layout_height="match_parent"
app:layout_behavior="@string/appbar_scrolling_view_behavior">
<androidx.appcompat.widget.LinearLayoutCompat
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<androidx.appcompat.widget.AppCompatTextView
android:layout_width="match_parent"
android:layout_height="200dp"
android:background="#FF0000" />
<androidx.appcompat.widget.AppCompatTextView
android:layout_width="match_parent"
android:layout_height="200dp"
android:background="#FF00FF" />
<androidx.appcompat.widget.AppCompatTextView
android:layout_width="match_parent"
android:layout_height="200dp"
android:background="#FF0000" />
<androidx.appcompat.widget.AppCompatTextView
android:layout_width="match_parent"
android:layout_height="200dp"
android:background="#FF00FF" />
<androidx.appcompat.widget.AppCompatTextView
android:layout_width="match_parent"
android:layout_height="200dp"
android:background="#FF0000" />
<androidx.appcompat.widget.AppCompatTextView
android:layout_width="match_parent"
android:layout_height="200dp"
android:background="#FF00FF" />
</androidx.appcompat.widget.LinearLayoutCompat>
</androidx.core.widget.NestedScrollView>
</androidx.coordinatorlayout.widget.CoordinatorLayout>
</layout>
MainActivity.kt
class MainActivity : AppCompatActivity() {
private lateinit var binding: ActivityMainBinding
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
binding = DataBindingUtil.setContentView(this, R.layout.activity_main)
binding.topAppBar.setNavigationOnClickListener {
Toast.makeText(this, "setNavigationOnClickListener", Toast.LENGTH_SHORT).show()
}
binding.topAppBar.setOnMenuItemClickListener { menuItem ->
when (menuItem.itemId) {
R.id.favorite -> {
Toast.makeText(this, "favorite", Toast.LENGTH_SHORT).show()
true
}
R.id.search -> {
Toast.makeText(this, "search", Toast.LENGTH_SHORT).show()
true
}
R.id.more -> {
Toast.makeText(this, "more", Toast.LENGTH_SHORT).show()
true
}
else -> false
}
}
}
}
2.Top app bar 滑動上抬標題與應用欄平行
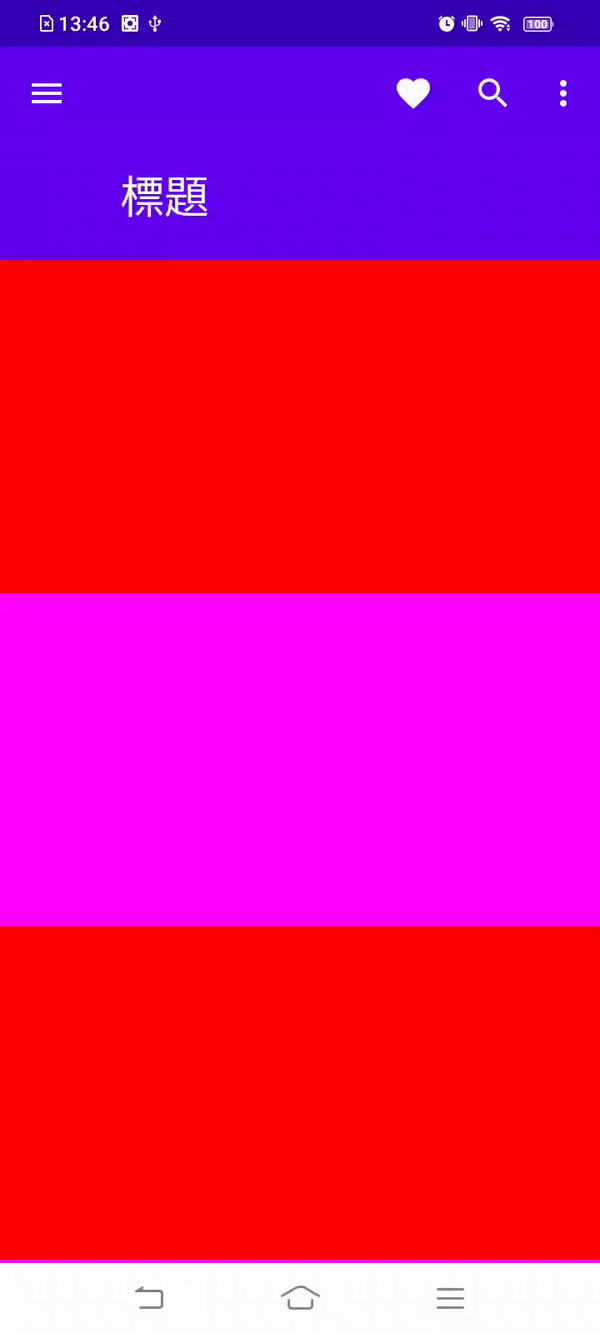
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<layout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools">
<data>
</data>
<androidx.coordinatorlayout.widget.CoordinatorLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<com.google.android.material.appbar.AppBarLayout
android:id="@+id/appBarLayout"
android:layout_width="match_parent"
android:layout_height="128dp">
<com.google.android.material.appbar.CollapsingToolbarLayout
app:layout_scrollFlags="scroll|exitUntilCollapsed|snap"
app:contentScrim="?attr/colorPrimary"
app:statusBarScrim="?attr/colorPrimaryVariant"
android:layout_width="match_parent"
android:layout_height="match_parent"
app:collapsedTitleTextAppearance="@style/TextAppearance.App.CollapsingToolbar.Collapsed"
app:expandedTitleMarginBottom="28dp"
app:expandedTitleMarginStart="72dp"
app:expandedTitleTextAppearance="@style/TextAppearance.App.CollapsingToolbar.Expanded">
<com.google.android.material.appbar.MaterialToolbar
app:layout_collapseMode="pin"
android:id="@+id/topAppBar"
style="@style/Widget.MaterialComponents.Toolbar.Primary"
android:layout_width="match_parent"
android:layout_height="?attr/actionBarSize"
android:elevation="0dp"
app:menu="@menu/top_app_bar"
app:navigationIcon="@drawable/ic_menu_24dp"
app:title="標題" />
</com.google.android.material.appbar.CollapsingToolbarLayout>
</com.google.android.material.appbar.AppBarLayout>
<androidx.core.widget.NestedScrollView
android:layout_width="match_parent"
android:layout_height="match_parent"
app:layout_behavior="@string/appbar_scrolling_view_behavior">
<androidx.appcompat.widget.LinearLayoutCompat
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<androidx.appcompat.widget.AppCompatTextView
android:layout_width="match_parent"
android:layout_height="200dp"
android:background="#FF0000" />
<androidx.appcompat.widget.AppCompatTextView
android:layout_width="match_parent"
android:layout_height="200dp"
android:background="#FF00FF" />
<androidx.appcompat.widget.AppCompatTextView
android:layout_width="match_parent"
android:layout_height="200dp"
android:background="#FF0000" />
<androidx.appcompat.widget.AppCompatTextView
android:layout_width="match_parent"
android:layout_height="200dp"
android:background="#FF00FF" />
<androidx.appcompat.widget.AppCompatTextView
android:layout_width="match_parent"
android:layout_height="200dp"
android:background="#FF0000" />
<androidx.appcompat.widget.AppCompatTextView
android:layout_width="match_parent"
android:layout_height="200dp"
android:background="#FF00FF" />
</androidx.appcompat.widget.LinearLayoutCompat>
</androidx.core.widget.NestedScrollView>
</androidx.coordinatorlayout.widget.CoordinatorLayout>
</layout>
3.Top app bar 滑動上抬標題與應用欄平行 + 圖片
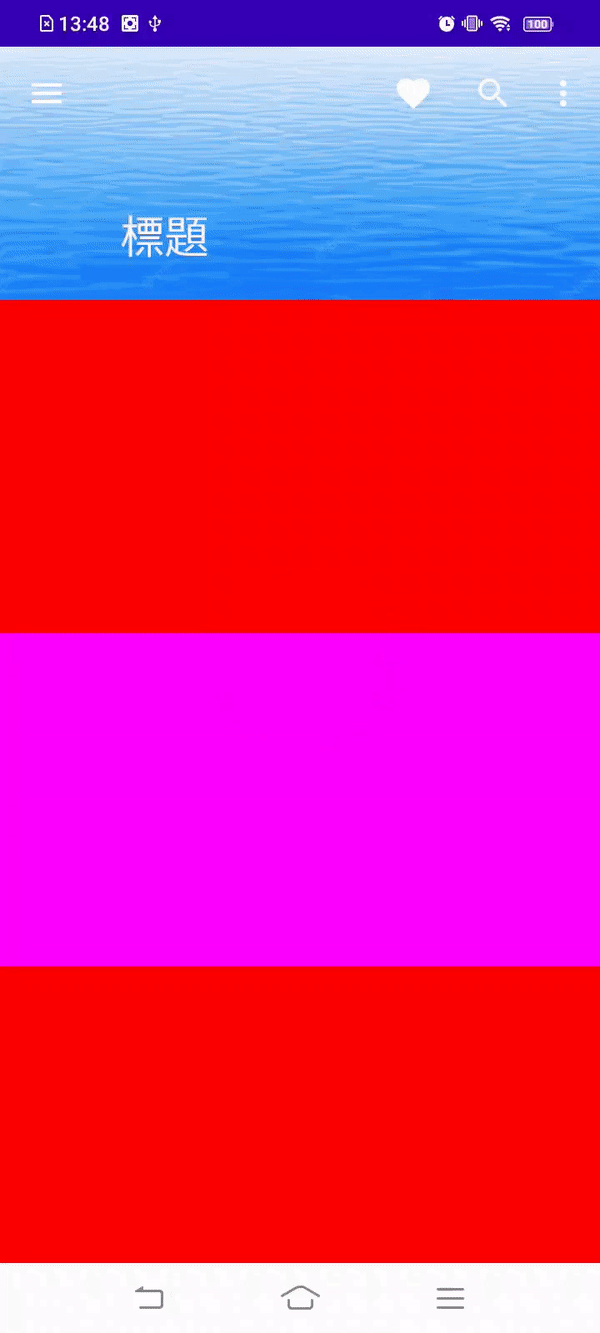
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<layout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools">
<data>
</data>
<androidx.coordinatorlayout.widget.CoordinatorLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<com.google.android.material.appbar.AppBarLayout
android:id="@+id/appBarLayout"
android:layout_width="match_parent"
android:layout_height="152dp">
<com.google.android.material.appbar.CollapsingToolbarLayout
app:layout_scrollFlags="scroll|exitUntilCollapsed|snap"
app:contentScrim="?attr/colorPrimary"
android:layout_width="match_parent"
android:layout_height="match_parent"
app:collapsedTitleTextAppearance="@style/TextAppearance.App.CollapsingToolbar.Collapsed"
app:expandedTitleMarginBottom="28dp"
app:expandedTitleMarginStart="72dp"
app:expandedTitleTextAppearance="@style/TextAppearance.App.CollapsingToolbar.Expanded">
<androidx.appcompat.widget.AppCompatImageView
android:layout_width="match_parent"
android:layout_height="match_parent"
android:src="@drawable/media"
android:scaleType="centerCrop" />
<com.google.android.material.appbar.MaterialToolbar
android:background="@android:color/transparent"
app:layout_collapseMode="pin"
android:id="@+id/topAppBar"
style="@style/Widget.MaterialComponents.Toolbar.Primary"
android:layout_width="match_parent"
android:layout_height="?attr/actionBarSize"
android:elevation="0dp"
app:menu="@menu/top_app_bar"
app:navigationIcon="@drawable/ic_menu_24dp"
app:title="標題" />
</com.google.android.material.appbar.CollapsingToolbarLayout>
</com.google.android.material.appbar.AppBarLayout>
<androidx.core.widget.NestedScrollView
android:layout_width="match_parent"
android:layout_height="match_parent"
app:layout_behavior="@string/appbar_scrolling_view_behavior">
<androidx.appcompat.widget.LinearLayoutCompat
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<androidx.appcompat.widget.AppCompatTextView
android:layout_width="match_parent"
android:layout_height="200dp"
android:background="#FF0000" />
<androidx.appcompat.widget.AppCompatTextView
android:layout_width="match_parent"
android:layout_height="200dp"
android:background="#FF00FF" />
<androidx.appcompat.widget.AppCompatTextView
android:layout_width="match_parent"
android:layout_height="200dp"
android:background="#FF0000" />
<androidx.appcompat.widget.AppCompatTextView
android:layout_width="match_parent"
android:layout_height="200dp"
android:background="#FF00FF" />
<androidx.appcompat.widget.AppCompatTextView
android:layout_width="match_parent"
android:layout_height="200dp"
android:background="#FF0000" />
<androidx.appcompat.widget.AppCompatTextView
android:layout_width="match_parent"
android:layout_height="200dp"
android:background="#FF00FF" />
</androidx.appcompat.widget.LinearLayoutCompat>
</androidx.core.widget.NestedScrollView>
</androidx.coordinatorlayout.widget.CoordinatorLayout>
</layout>
4.Top app bar 操作欄
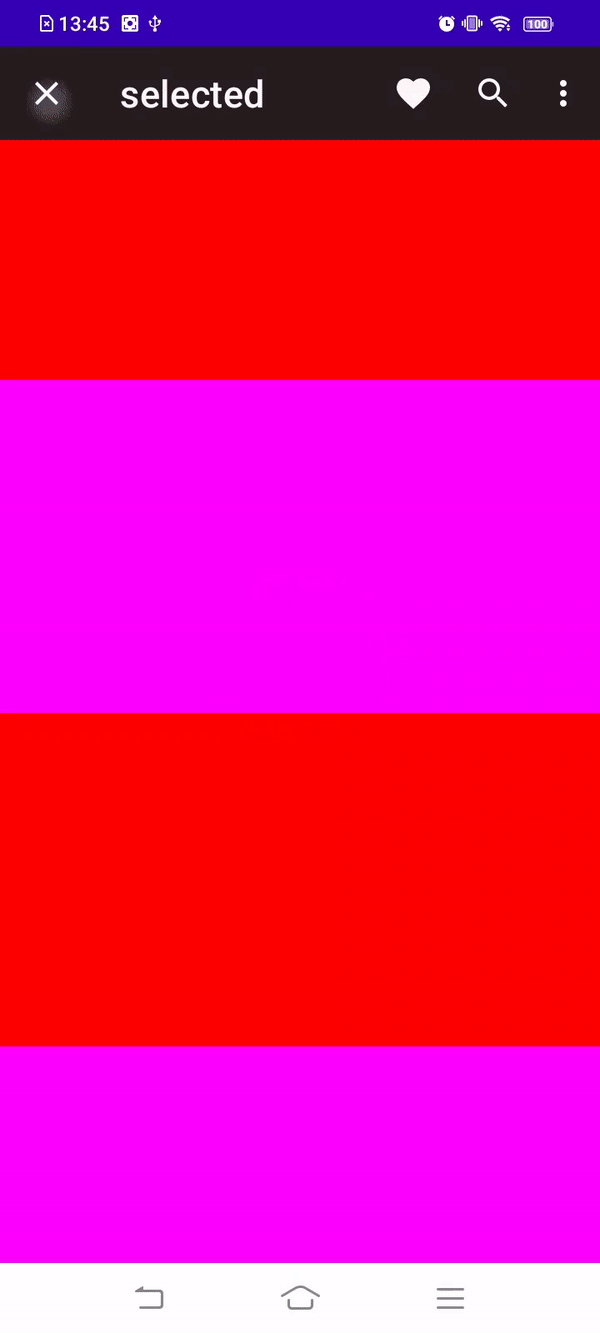
acitivty_main.xml
<?xml version="1.0" encoding="utf-8"?>
<layout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools">
<data>
</data>
<androidx.coordinatorlayout.widget.CoordinatorLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<androidx.core.widget.NestedScrollView
android:layout_width="match_parent"
android:layout_height="match_parent"
app:layout_behavior="@string/appbar_scrolling_view_behavior">
<androidx.appcompat.widget.LinearLayoutCompat
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<androidx.appcompat.widget.AppCompatTextView
android:id="@+id/a"
android:layout_width="match_parent"
android:layout_height="200dp"
android:background="#FF0000" />
<androidx.appcompat.widget.AppCompatTextView
android:layout_width="match_parent"
android:layout_height="200dp"
android:background="#FF00FF" />
<androidx.appcompat.widget.AppCompatTextView
android:layout_width="match_parent"
android:layout_height="200dp"
android:background="#FF0000" />
<androidx.appcompat.widget.AppCompatTextView
android:layout_width="match_parent"
android:layout_height="200dp"
android:background="#FF00FF" />
<androidx.appcompat.widget.AppCompatTextView
android:layout_width="match_parent"
android:layout_height="200dp"
android:background="#FF0000" />
<androidx.appcompat.widget.AppCompatTextView
android:layout_width="match_parent"
android:layout_height="200dp"
android:background="#FF00FF" />
</androidx.appcompat.widget.LinearLayoutCompat>
</androidx.core.widget.NestedScrollView>
</androidx.coordinatorlayout.widget.CoordinatorLayout>
</layout>
MainActivity.kt
class MainActivity : AppCompatActivity() {
private lateinit var binding: ActivityMainBinding
private var actionMode: ActionMode? = null
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
binding = DataBindingUtil.setContentView(this, R.layout.activity_main)
val callback = object : ActionMode.Callback {
override fun onCreateActionMode(mode: ActionMode?, menu: Menu?): Boolean {
menuInflater.inflate(R.menu.top_app_bar, menu)
return true
}
override fun onPrepareActionMode(mode: ActionMode?, menu: Menu?): Boolean {
return false
}
override fun onActionItemClicked(mode: ActionMode?, item: MenuItem?): Boolean {
return when (item?.itemId) {
R.id.favorite -> {
Toast.makeText(this@MainActivity, "favorite", Toast.LENGTH_SHORT).show()
true
}
R.id.search -> {
Toast.makeText(this@MainActivity, "search", Toast.LENGTH_SHORT).show()
true
}
R.id.more -> {
Toast.makeText(this@MainActivity, "more", Toast.LENGTH_SHORT).show()
true
}
else -> false
}
}
override fun onDestroyActionMode(mode: ActionMode?) {
Toast.makeText(this@MainActivity, "onDestroyActionMode", Toast.LENGTH_SHORT).show()
actionMode = null
}
}
binding.a.setOnClickListener {
if (actionMode == null) {
actionMode = startSupportActionMode(callback)
actionMode?.title = "selected"
}
}
}
}
5.Developer Documents Top app bar
Open in Documents Top app bar